python package 생성
pip는 Python Package Index(PyPI)를 일컷는 약자로 파이썬 패키지를 관리하는 패키지 저장소이다.
우선 단계를 구분하자면
0. pypi 계정 생성, git 계정 생성
1. github repository 생성
2. 사용할 함수 및 폴더 구성
3. setup.py, __init__.py, README.md, setup.cfg, .gitignore, git init 생성
4. 등록
5. 사용
1. github repository
github 들어가서 아래처럼 Repository를 생성한다. 가급적 이름은 패키지랑 동일한게 좋으나 달라도 무방하다.
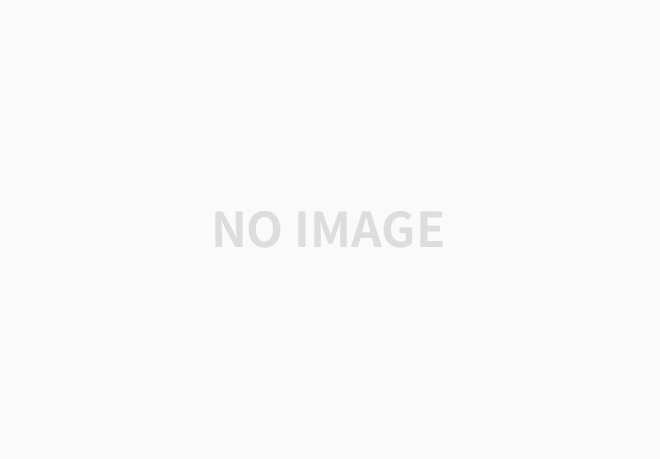
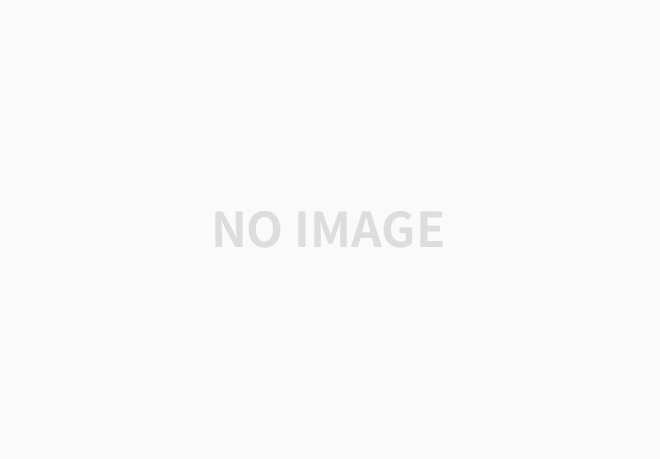
pca_cj 가 생성할 package name이며, 사용할 함수 및 폴더 구성은 다음과 같다.
<package name>
ㄴ<package name>
ㄴfunction1.py
ㄴfunction2.py
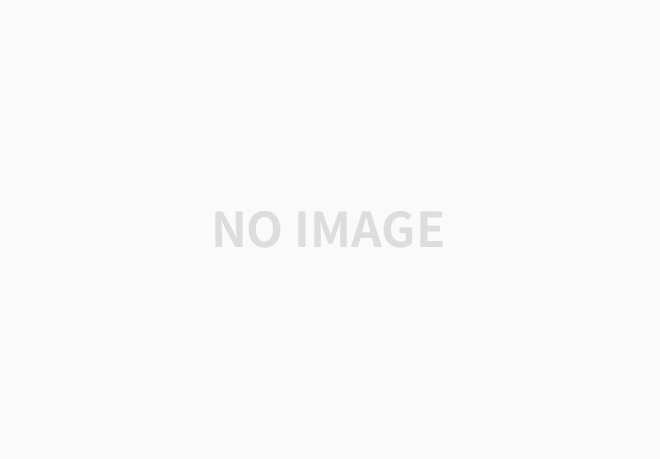
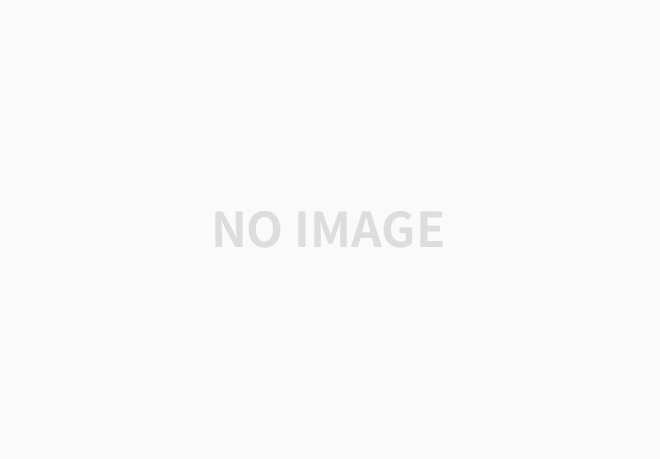
setup.py
from setuptools import setup, find_packages
setup(
# 배포할 패키지의 이름을 적어줍니다. setup.py파일을 가지는 폴더 이름과 동일하게 합니다.
name = 'pca_cj',
# 배포할 패키지의 버전을 적어줍니다. 첫 등록이므로 0.1 또는 0.0.1을 사용합니다.
version = '0.2',
# 배포할 패키지에 대한 설명을 작성합니다.
description = 'cor pca method generate',
# 배포하는 사람의 이름을 작성합니다.
author = 'changje.cho',
# 배포하는 사람의 메일주소를 작성합니다.
author_email = 'qkdrk7777775@gmail.com',
# 배포하는 패키지의 url을 적어줍니다. 보통 github 링크를 적습니다.
url = 'https://github.com/qkdrk7777775/pca_cj',
# 배포하는 패키지의 다운로드 url을 적어줍니다.
download_url = 'https://github.com/qkdrk7777775/pca_cj/archive/master.zip',
# 해당 패키지를 사용하기 위해 필요한 패키지를 적어줍니다. ex. install_requires= ['numpy', 'django']
# 여기에 적어준 패키지는 현재 패키지를 install할때 함께 install됩니다.
install_requires = [],
# 등록하고자 하는 패키지를 적는 곳입니다.
# 우리는 find_packages 라이브러리를 이용하기 때문에 아래와 같이 적어줍니다.
# 만약 제외하고자 하는 파일이 있다면 exclude에 적어줍니다.
packages = find_packages(exclude = []),
# 패키지의 키워드를 적습니다.
keywords = ['correlation matrix principal component analysis'],
# 해당 패키지를 사용하기 위해 필요한 파이썬 버전을 적습니다.
python_requires = '>=3',
# 파이썬 파일이 아닌 다른 파일을 포함시키고 싶다면 package_data에 포함시켜야 합니다.
package_data = {},
# 위의 package_data에 대한 설정을 하였다면 zip_safe설정도 해주어야 합니다.
zip_safe = False,
# PyPI에 등록될 메타 데이터를 설정합니다.
# 이는 단순히 PyPI에 등록되는 메타 데이터일 뿐이고, 실제 빌드에는 영향을 주지 않습니다.
classifiers = [
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.2',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
],
)
__init__.py : 내용은 없어도 무방하나 없으면 패키지가 설치가 안됨.
README.md : github에서 보여질 문서
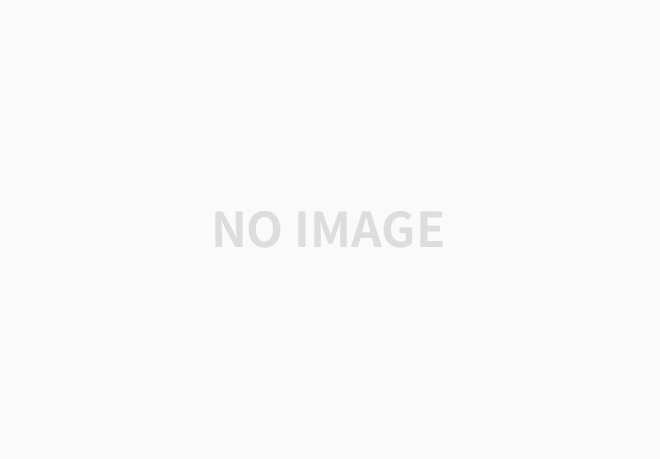
# This Package is correlation matrix principal component analysis.
## package example1
cor pca use iris data
```
from sklearn.datasets import load_iris
import pandas as pd
df= load_iris()
df=pd.concat([pd.DataFrame(df.data),pd.Series(df.target)],axis=1)
df.columns=['sepal_len', 'sepal_wid', 'petal_len', 'petal_wid', 'class']
df.tail()
X = df.iloc[:,0:4].values
y = df.iloc[:,4].values
#sklearn default is PCA(X,cor=False,cov_scaling=True,center=True)
finelDF,mean,std,matrix_w=PCA(X,cor=False,cov_scaling=False)
finelDF,mean,std,matrix_w=PCA(X,cor=True)
from matplotlib import pyplot as plt
fig = plt.figure(figsize = (8,8))
ax = fig.add_subplot(1,1,1)
ax.set_xlabel('Principal Component 1', fontsize = 15)
ax.set_ylabel('Principal Component 2', fontsize = 15)
ax.set_title('2 component PCA', fontsize = 20)
targets = [2, 1, 0]
colors = ['r', 'g', 'b']
for target, color in zip(targets,colors):
indicesToKeep = y== target
ax.scatter(finelDF[indicesToKeep ,0]
, finelDF[indicesToKeep ,1]
, c = color
, s = 50)
ax.legend(targets)
ax.grid()
#pca prediction
a=PCA(X[1:50],cor=False,cov_scaling=False)
#prediction
pred=(X[51:,:]-a[1]).dot(a[3])
```
## package example2
```
#sklearn kernel PCA method but scale is diffrence
X, y = make_moons(n_samples=100, random_state=123)
X_skernpca ,lambdas= kernel_PCA(X,n_components=2, gamma=15,scaling=False)
plt.scatter(X_skernpca[y == 0, 0], X_skernpca[y == 0, 1],
color='red', marker='^', alpha=0.5)
plt.scatter(X_skernpca[y == 1, 0], X_skernpca[y == 1, 1],
color='blue', marker='o', alpha=0.5)
plt.xlabel('PC1')
plt.ylabel('PC2')
plt.tight_layout()
plt.show()
```
setup.cfg
[metadata]
description-file = README.md
.gitignore : 깃 등록시 무시되게 설정해주는 파일.
# vscode
.vscode/
# Byte-compiled / optimized / DLL files
__pycache__/
*.py[cod]
*$py.class
# C extensions
*.so
# Distribution / packaging
.Python
build/
develop-eggs/
dist/
downloads/
eggs/
.eggs/
lib/
lib64/
parts/
sdist/
var/
wheels/
pip-wheel-metadata/
share/python-wheels/
*.egg-info/
.installed.cfg
*.egg
MANIFEST
# PyInstaller
# Usually these files are written by a python script from a template
# before PyInstaller builds the exe, so as to inject date/other infos into it.
*.manifest
*.spec
# Installer logs
pip-log.txt
pip-delete-this-directory.txt
# Unit test / coverage reports
htmlcov/
.tox/
.nox/
.coverage
.coverage.*
.cache
nosetests.xml
coverage.xml
*.cover
.hypothesis/
.pytest_cache/
# Translations
*.mo
*.pot
# Django stuff:
*.log
local_settings.py
db.sqlite3
# Flask stuff:
instance/
.webassets-cache
# Scrapy stuff:
.scrapy
# Sphinx documentation
docs/_build/
# PyBuilder
target/
# Jupyter Notebook
.ipynb_checkpoints
# IPython
profile_default/
ipython_config.py
# pyenv
.python-version
# celery beat schedule file
celerybeat-schedule
# SageMath parsed files
*.sage.py
# Environments
.env
.venv
env/
venv/
ENV/
env.bak/
venv.bak/
# Spyder project settings
.spyderproject
.spyproject
# Rope project settings
.ropeproject
# mkdocs documentation
/site
# mypy
.mypy_cache/
.dmypy.json
dmypy.json
# Pyre type checker
.pyre/
git init
git add .
git commit -m 'repo init'
git remote add origin http://github.com/<깃계정>/<패키지명>.git
git push -u origin master
패키지 등록을 위해 3가지 패키지가 요구
setuptools, wheel, twine
pip install setuptools wheel twine
python setup.py bdist_wheel
명령어 실행시 파일이 생성 되는데 dist 경로를 확인
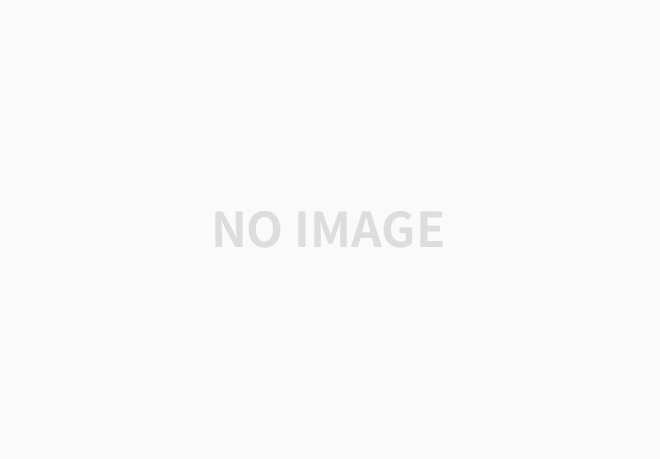
twine upload dist/pca_cj-0.2-py3-none-any.whl
이제 pip install pca_cj로 다운로드 받아 실행하면되며,
python에서는
from pca_cj import <function명>의 형태로 사용하면 된다.
만약 도중에
fatal: remote origin already exists 란 애러가 발생할 시
git remote rm origin 를 사용하여 remote origin을 제거하고 다시 진행하면 설치 될 것이다.
참고 : https://doorbw.tistory.com/225
파이썬(PYTHON) #25_ 파이썬 패키지 등록하기 (pip 배포하기)
안녕하세요. 문범우입니다. 이번 포스팅에서는 파이썬 패키지를 배포하는 방법에 대해서 함께 살펴보도록 하겠습니다. 1. pip: 파이썬 패키지 관리자 파이썬 패키지를 배포하는 방법에 대해 설명
doorbw.tistory.com